Server authentication
When configuring webhooks for your assistant, you can authenticate your server endpoints using either a secret token, custom headers, or OAuth2. This ensures that only authorized requests from Vapi are processed by your server.
Credential Configuration
Credentials can be configured at multiple levels:
- Tool Call Level: Create individual credentials for each tool call
- Assistant Level: Set credentials directly in the assistant configuration
- Phone Number Level: Configure credentials for specific phone numbers
- Organization Level: Manage credentials in the API Keys page
The order of precedence is:
- Tool call-level credentials
- Assistant-level credentials
- Phone number-level credentials
- Organization-level credentials from the API Keys page
Authentication Methods
Secret Token Authentication
The simplest way to authenticate webhook requests is using a secret token. Vapi will include this token in the X-Vapi-Signature
header of each request.
Configuration
Custom Headers Authentication
For more complex authentication scenarios, you can configure custom headers that Vapi will include with each webhook request.
This could include short lived JWTs/API Keys passed along via the Authorization header, or any other header that your server checks for.
Configuration
OAuth2 Authentication
For OAuth2-protected webhook endpoints, you can configure OAuth2 credentials that Vapi will use to obtain and refresh access tokens.
Configuration (at the assistant-level)
Configuration (via our Dashboard)
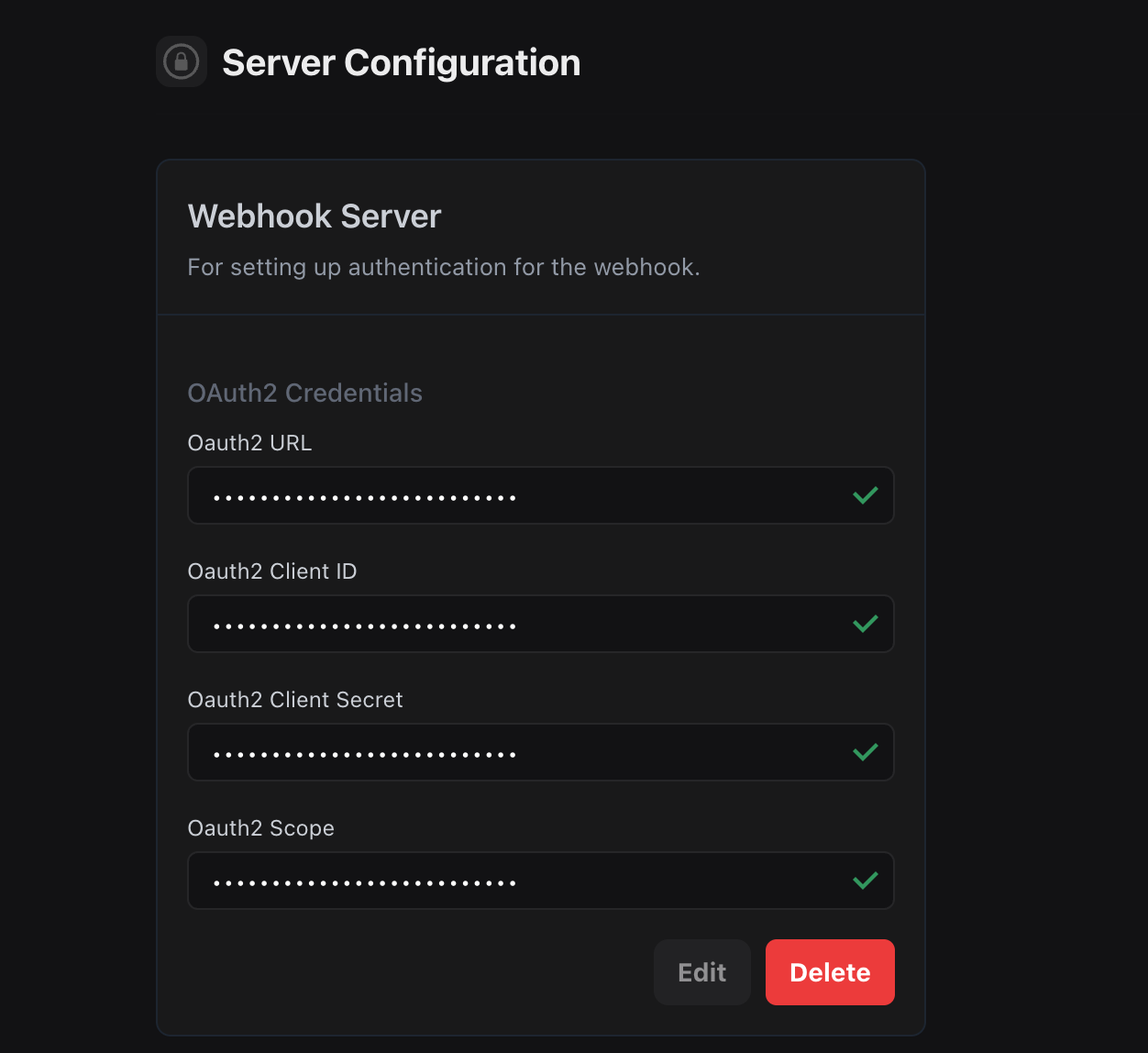
OAuth2 Flow
- Vapi makes a request to your token endpoint with client credentials (Content-Type
application/x-www-form-urlencoded
) - Your server validates the credentials and returns an access token
- Vapi includes the access token in the Authorization header for webhook requests
- Your server validates the access token before processing the webhook
- When the token expires, Vapi automatically requests a new one
OAuth2 Token Response Format
Your server should return a JSON response with the following format:
Example error response:
Common error types:
invalid_client
: Invalid client credentialsinvalid_grant
: Invalid or expired refresh tokeninvalid_scope
: Invalid scope requestedunauthorized_client
: Client not authorized for this grant type