Lead qualification workflow
Overview
Build an AI-powered outbound sales workflow that qualifies leads, handles objections, and schedules appointments using Vapi workflows with sophisticated branching logic and CRM integration.
What You’ll Build:
- Lead qualification with BANT scoring and conditional routing
- Objection handling with global nodes and sentiment analysis
- Appointment scheduling with calendar integration
- CRM updates with automated follow-up sequences
Prerequisites
- A Vapi account.
- A CRM system or customer database.
- A calendar system for appointment scheduling.
Scenario
We will be creating an outbound sales workflow for TechFlow Solutions, a B2B software company that wants to automate their lead qualification process with sophisticated branching logic to handle different prospect scenarios and increase appointment booking rates.
Final Workflow
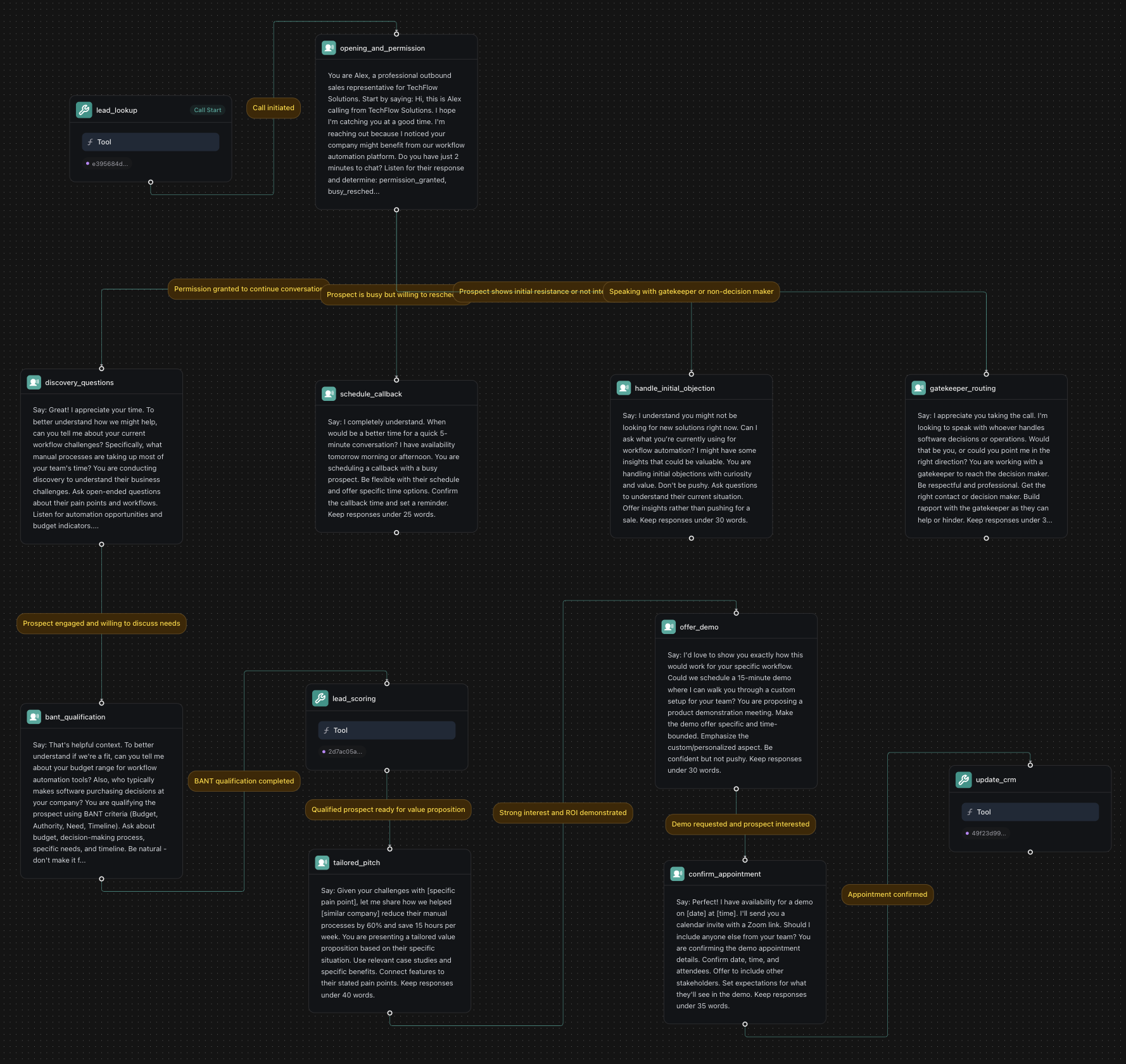
1. Create a Knowledge Base
2. Create Required Tools
Before building the workflow, create the necessary tools in your dashboard:
Create Lead Lookup Tool
Click Create Tool
and configure:
- Tool Name: “Lead Lookup”
- Tool Type: “Function”
- Function Name:
lookup_lead
- Description: “Retrieve lead information and call history”
- Parameters:
lead_id
(string): Lead ID to lookup
- Server URL:
https://jsonplaceholder.typicode.com/users
This example uses JSONPlaceholder for demonstration. In production, replace with your CRM API (Salesforce, HubSpot, etc.).
Create Lead Scoring Tool
Create another tool:
- Tool Name: “Lead Scoring”
- Function Name:
score_lead
- Description: “Score leads based on qualification responses”
- Parameters:
budget
(string): Budget informationauthority
(string): Decision-making authorityneed
(string): Business need assessmenttimeline
(string): Purchase timeline
- Server URL:
https://jsonplaceholder.typicode.com/posts
This example uses JSONPlaceholder for demonstration. Replace with your lead scoring system in production.
Create CRM Update Tool
Create a third tool:
- Tool Name: “CRM Update”
- Function Name:
update_crm
- Description: “Update CRM with call outcomes and next steps”
- Parameters:
lead_id
(string): Lead identifiercall_outcome
(string): Result of the callnext_steps
(string): Planned follow-up actions
- Server URL:
https://jsonplaceholder.typicode.com/posts
This example uses JSONPlaceholder for demonstration. In production, integrate with your CRM system.
3. Create a Workflow
4. Build the Workflow
You’ll start with a default template that includes a “Call Start” node. We’ll modify the existing nodes and add new ones to create our outbound sales workflow.
Configure the Initial Conversation Node
The default template includes a conversation node. Click on it and configure:
Node Name: opening_and_permission
Extract Variables:
- Variable:
permission_status
- Type:
String
- Description:
The prospect's response to the initial request
- Enum Values:
permission_granted
,busy_reschedule
,not_interested
,gatekeeper
Add Lead Lookup Tool Node
Add a Tool node that runs before the opening:
Tool: Select your pre-configured “Lead Lookup” tool from the dropdown. This tool should be created in the Tools section of your dashboard with:
- Function Name:
lookup_lead
- Description: “Retrieve lead information and call history”
- Parameters:
lead_id
(string): Lead ID to lookup
- Server URL:
https://jsonplaceholder.typicode.com/users
Add Permission-Based Routing
Create branching paths based on the prospect’s response. Add multiple conversation nodes:
Discovery Questions Node:
Node Name: discovery_questions
Reschedule Node:
Node Name: schedule_callback
Objection Handling Node:
Node Name: handle_initial_objection
Gatekeeper Node:
Node Name: gatekeeper_routing
Configure Flow Conditions
Connect the nodes with conditions for the LLM to interpret:
To Discovery Questions Node:
- Condition:
Permission granted to continue conversation
To Reschedule Node:
- Condition:
Prospect is busy but willing to reschedule
To Objection Handling Node:
- Condition:
Prospect shows initial resistance or not interested
To Gatekeeper Node:
- Condition:
Speaking with gatekeeper or non-decision maker
Add Global Objection Handler
Create a global node that handles objections throughout the call:
Node Name: objection_handler
Global Node: enabled = true
Enter Condition: {{ objection_detected == true or negative_sentiment == true }}
This global node will activate whenever an objection is detected, regardless of where they are in the sales conversation.
Add Qualification Flow
For prospects who engage, add these qualification nodes:
BANT Qualification Node:
Node Name: bant_qualification
Extract Variables:
- Variable:
budget_range
- Type:
String
- Description:
Budget information provided
- Variable:
decision_authority
- Type:
String
- Description:
Decision-making authority level
- Variable:
timeline
- Type:
String
- Description:
Purchase timeline or urgency
Lead Scoring Tool Node:
- Add a Tool node that calls lead scoring API based on qualification responses
Qualification Results Node:
Node Name: route_by_score
- Route based on lead score (hot, warm, cold)
Add Value Proposition Flow
Industry-Specific Pitch Node:
Node Name: tailored_pitch
- Present value proposition based on their industry and pain points
ROI Demonstration Node:
Node Name: show_roi
- Provide specific ROI examples and case studies
Demo Offer Node:
Node Name: offer_demo
- Propose a product demonstration meeting
Add Appointment Scheduling Flow
Calendar Check Tool Node:
- Add a Tool node that checks sales team calendar for available demo slots
Appointment Confirmation Node:
Node Name: confirm_appointment
- Confirm meeting details and send calendar invite
CRM Update Tool Node:
- Add a Tool node that records call outcome and next steps in CRM
Add Transfer and Follow-up Options
Transfer to Sales Rep Node:
Node Type: Transfer
Destination: +1-555-SALES-1
(your sales team number)
Schedule Follow-up Node:
Node Name: schedule_followup
- Set automated follow-up call for future date
End Call Node:
Node Type: Hangup
- Use when prospect is not qualified or requests no further contact
Integrating with Real Systems
This example uses JSONPlaceholder for demonstration purposes. To integrate with your actual sales systems:
CRM Platform Integration
- Salesforce: Use the Salesforce REST API
- HubSpot: Use the HubSpot API
- Pipedrive: Use the Pipedrive API
Calendar Integration
- Google Calendar: Google Calendar API
- Microsoft Outlook: Microsoft Graph API
- Calendly: Calendly API
Communication Tools
- Twilio: Twilio API for SMS and voice
- SendGrid: SendGrid API for email
- Slack: Slack API for team notifications
Next Steps
Just like that, you’ve built an outbound sales qualification workflow that can handle lead qualification, objection handling, and appointment scheduling with automated CRM integration.
Consider reading the following guides to further enhance your workflow:
- Custom Tools - Create custom tools for CRM integration and lead management.
- Voice Formatting Plan - Configure speech patterns for professional sales conversations.
- Call Analysis - Analyze call performance and optimize sales conversations.